StatevectorEstimator
class qiskit.primitives.StatevectorEstimator(*, default_precision=0.0, seed=None)
Bases: BaseEstimatorV2
Simple implementation of BaseEstimatorV2
with full state vector simulation.
This class is implemented via Statevector
which turns provided circuits into pure state vectors. These states are subsequently acted on by :class:~.SparsePauliOp`, which implies that, at present, this implementation is only compatible with Pauli-based observables.
Each tuple of (circuit, observables, <optional> parameter values, <optional> precision)
, called an estimator primitive unified bloc (PUB), produces its own array-based result. The run()
method can be given a sequence of pubs to run in one call.
from qiskit.circuit import Parameter, QuantumCircuit
from qiskit.primitives import StatevectorEstimator
from qiskit.quantum_info import Pauli, SparsePauliOp
import matplotlib.pyplot as plt
import numpy as np
# Define a circuit with two parameters.
circuit = QuantumCircuit(2)
circuit.h(0)
circuit.cx(0, 1)
circuit.ry(Parameter("a"), 0)
circuit.rz(Parameter("b"), 0)
circuit.cx(0, 1)
circuit.h(0)
# Define a sweep over parameter values, where the second axis is over
# the two parameters in the circuit.
params = np.vstack([
np.linspace(-np.pi, np.pi, 100),
np.linspace(-4 * np.pi, 4 * np.pi, 100)
]).T
# Define three observables. Many formats are supported here including
# classes such as qiskit.quantum_info.SparsePauliOp. The inner length-1
# lists cause this array of observables to have shape (3, 1), rather
# than shape (3,) if they were omitted.
observables = [
[SparsePauliOp(["XX", "IY"], [0.5, 0.5])],
[Pauli("XX")],
[Pauli("IY")]
]
# Instantiate a new statevector simulation based estimator object.
estimator = StatevectorEstimator()
# Estimate the expectation value for all 300 combinations of
# observables and parameter values, where the pub result will have
# shape (3, 100). This shape is due to our array of parameter
# bindings having shape (100,), combined with our array of observables
# having shape (3, 1)
pub = (circuit, observables, params)
job = estimator.run([pub])
# Extract the result for the 0th pub (this example only has one pub).
result = job.result()[0]
# Error-bar information is also available, but the error is 0
# for this StatevectorEstimator.
result.data.stds
# Pull out the array-based expectation value estimate data from the
# result and plot a trace for each observable.
for idx, pauli in enumerate(observables):
plt.plot(result.data.evs[idx], label=pauli)
plt.legend()
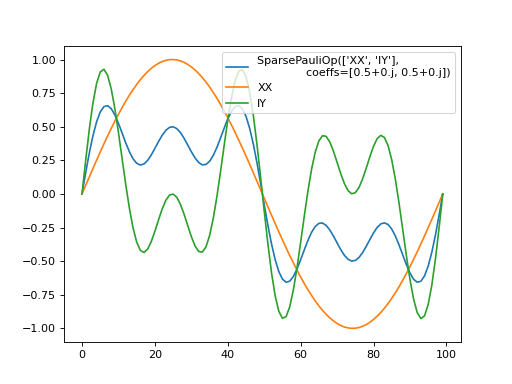
Parameters
- default_precision (float(opens in a new tab)) – The default precision for the estimator if not specified during run.
- seed (np.random.Generator | int(opens in a new tab) | None) – The seed or Generator object for random number generation. If None, a random seeded default RNG will be used.
Attributes
default_precision
Return the default precision
seed
Return the seed or Generator object for random number generation.
Methods
run
run(pubs, *, precision=None)
Estimate expectation values for each provided pub (Primitive Unified Bloc).
Parameters
- pubs (Iterable[EstimatorPubLike]) – An iterable of pub-like objects, such as tuples
(circuit, observables)
or(circuit, observables, parameter_values)
. - precision (float(opens in a new tab) | None) – The target precision for expectation value estimates of each run Estimator Pub that does not specify its own precision. If None the estimator’s default precision value will be used.
Returns
A job object that contains results.
Return type